Cart Limits
Disable quantity button in the Cart when TryNow limit has been met.
What are Cart Limits?
Cart Limits are set in the TryNow Merchant Portal where they can also be modified. They are values set in Merchant Portal to define how many items a Shopper can try before buying.
Prerequisites
In order to use Cart Limits, you should have a working TryNow implementation with a functioning button on your product detail page. Cart Limits should be set within the TryNow Merchant Portal.
Implementation
In a successful implementation, the quantity buttons will present a tooltip and be disabled based on whether or not the Cart Limit is reached.
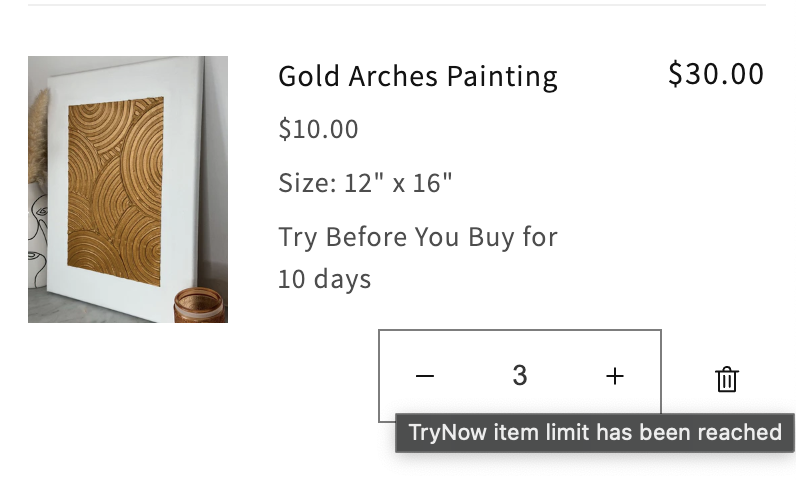
The quantity button in a cart will not automatically be disabled when the TryNow Cart Limit is met. TryNow exposes an asynchronous method on the window.trynow.cart
object isTryNowCartLimitMet()
that takes no arguments and returns a boolean where true
indicates the TryNow items in the Cart have reached the Cart Limits.
const isCartLimitMet = await window.trynow.cart.isTryNowCartLimitMet();
You can use this method to disable the quantity buttons via Javascript. More resources on methods exposed on the Cart object here . The following steps can be followed to disable the quantity button when the Cart Limit is met for TryNow items:
Disabling the Quantity Button
Disable on Page Load
The following code will disable quantity buttons only on DOMContentLoaded
. To listen to the DOM for changes and update the disablement, use a MutationObserver.
-
Locate where the quantity button is in the code and add a TryNow
id
orclass
to it if the item is on a TryNow selling plan. This quantity button will exist in the{% for item in cart.items %}
loop if your minicart is built with Liquid. In the case of a Javascript built minicart, look for the button inside the loop that renders you cart items:<button class="quantity_button no-js-hidden {% if item.selling_plan_allocation and item.selling_plan_allocation.selling_plan.name contains 'Try Before You Buy' %} TryNow-Item {% endif %} " name="plus" type="button" >
-
In that same template, add the following JavaScript somewhere on the page, like a
<script>
tag inside your<head>
:document.addEventListener("DOMContentLoaded", async function () { const isCartLimitMet = await window.trynow.cart.isTryNowCartLimitMet(); if (!isCartLimitMet) return; const quantityButtons = document.querySelectorAll(".TryNow-Item"); if (!quantityButtons) { console.info("Element with Class TryNow-Item not found."); return; } quantityButtons.forEach((quantityButton) => { quantityButton.disabled = true; quantityButton.title = "TryNow item limit has been reached"; }); });
Disable Using Observers
Disabling quantity buttons based on changes in the DOM can be preferable to provide the Shopper with the best possible TryNow experience. That is, if a Shopper adds an item to the cart which does not trigger a page reload, an observer will be required to disable the quantity buttons.
Mutation Observers
For more information regarding Mutation Observers and their impacts, please refer to the MDN WebDocs.
Using Mutation Observers, you can listen for changes on the DOM to conditionally hide or disable the quantity buttons in the cart when the TryNow Cart Limit upper bound is met.
The simplest method is to add a class to TryNow line item quantity buttons/elements as mentioned in step #1 of disabling on page load, and disable them when DOM changes are observed and the Cart Limit is met.
const observer = new MutationObserver((mutationsList) => {
mutationsList.forEach(async (record) => {
if (!window.trynow) return;
const upperLimitIsMet = await window.trynow.cart.isTryNowCartLimitMet();
if (record.target && upperLimitIsMet) {
const quantityButtonNodes = document.querySelectorAll(
".TryNow-Item-QuantityButton",
);
quantityButtonNodes.forEach((btn) => {
btn.disabled = true;
btn.title = "TryNow item limit has been reached";
});
}
});
});
const cartDrawer = document.querySelector("#CartDrawer");
observer.observe(cartDrawer, {
subtree: true,
childList: true,
});
Troubleshooting
Quantity Button Does Not Disable
If the quantity button in the cart's markup is not a <button>
element you won't be able to disable it using the disabled attribute.
Instead, CSS properties can be used to make the element un-clickable and un-selectable, such as pointer-events: none
. Keep in mind this will eliminate all pointer events, so any tooltips or hover behavior will be lost.
Updated 2 months ago