Adjusted Line Item Prices
Display the price of TryNow items as $0 in the cart.
What are Adjusted Line Item Prices?
Typically, the line items in the cart display the amount if kept price. Sometimes this accounts for the quantity of items. However for products added with the TryNow selling plan, the total price should always be shown as $0.
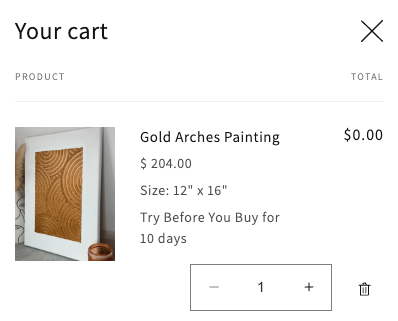
Prerequisites
You must be able to add Try Before You Buy items to the cart.
Implementing the Adjusted Prices
Liquid
In some cases, it will be enough to make changes directly in the Liquid template of the cart or side cart.
- Locate the element that renders the price. It may look like this:
<span class="price price--end">
{{ item.original_line_price | money }}
</span>
- Add logic to render either $0 or the actual price based on the presence of the TryNow selling plan in the item.
<span class="price price--end">
{% if item.selling_plan_allocation.selling_plan.name contains 'Try' %}
{{ 0 | money }}
{% else %}
{{ item.original_line_price | money }}
{% endif %}
</span>
After implementing this code, the result will be:
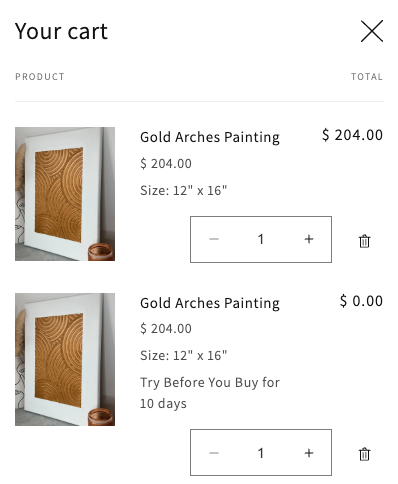
JavaScript
There are two ways to implement adjusted prices in JavaScript:
- Modify the JavaScript code in your theme to ensure the correct price is rendered using logic similar to the previous examples.
- Use Mutation Observers to detect changes in the cart and make the necessary adjustments.
The Cart Items Loop
Universally applicable code can be challenging because each Shopify theme is unique which leads to variation. The following acts as a guideline but will vary depending on your theme.
- Find the function responsible for rendering the Cart.
- Within that function, look for the section where cart items are processed. This section is typically a loop that iterates through all items in the cart.
- Before rendering the price for each item within the loop, check if the item includes the "Try Before You Buy" selling plan.
items.forEach(item => {
const hasTryPlan = item.selling_plan_allocation?.some(allocation =>
allocation.selling_plan?.name.includes('Try Before You Buy')
);
let priceElement;
if (hasTryPlan) {
priceElement = `<div class="price">$0.00</div>`;
} else {
priceElement = `<div class="price">${item.line_price}</div>`;
}
});
Mutation Observers
In order to track changes to the cart, you may need to implement a Mutation Observer.
- Identify the Cart Template and insert script tags.
<script type="text/javascript">
</script>
- Store the Cart container in a variable.
const cart = document.querySelector('[CART_CONTAINER]')
- Create a Mutation Observer if one does not already exist.
const cartObserver = new MutationObserver(() => {
})
cartObserver.observe(cart, {
subtree: true,
childList: true,
})
- Within the Mutation Observer, implement logic to identify TryNow items in the cart and update the price if the item is associated with the Try Before You Buy selling plan.
const tryItems = Array.from(cart.querySelectorAll('[CART_ITEM_SELECTOR]')).filter(item => {
const productOption = item.querySelector('[TRYNOW_LABEL_SELECTOR]');
return productOption && productOption.textContent.includes('Try Before You Buy');
});
tryItems.forEach(item => {
item.querySelector('[ITEM_PRICE_SELECTOR]').textContent = '$0.00'
})
- The completed
<script>
may appear as follows:
<script type="text/javascript">
const cart = document.querySelector('[CART_CONTAINER]')
const cartObserver = new MutationObserver(() => {
const tryItems = Array.from(cart.querySelectorAll('[CART_ITEM_SELECTOR]')).filter(item => {
const productOption = item.querySelector('[TRYNOW_LABEL_SELECTOR]');
return productOption && productOption.textContent.includes('Try');
});
tryItems.forEach(item => {
item.querySelector('[ITEM_PRICE_SELECTOR]').textContent = '$0.00'
})
})
cartObserver.observe(cart, {
subtree: true,
childList: true,
})
</script>
<script type="text/javascript">
const cart = document.querySelector('[CART_CONTAINER]')
const cartObserver = new MutationObserver(() => {
const tryItems = Array.from(cart.querySelectorAll('[CART_ITEM_SELECTOR]')).filter(item => {
const productOption = item.querySelector('[TRYNOW_LABEL_SELECTOR]');
return productOption && productOption.textContent.includes('Try');
});
tryItems.forEach(item => {
item.querySelector('[ITEM_PRICE_SELECTOR]').textContent = '$0.00'
})
})
cartObserver.observe(cart, {
subtree: true,
childList: true,
})
</script>
Updated 17 days ago