Adjusted Subtotals
Purchasing items using TryNow changes the amount due at checkout subtotal.
What are Adjusted Subtotals?
Typical subtotal displays with Shopify don't properly handle the presence of Try Before You Buy items in a Shopper's cart. Updating the subtotal value in your cart to reflect an accurate Amount Due At Checkout value can improve the Shopper experience. This can be done using Liquid and/or Javascript to display correct amounts.
For example, in this test store cart we have one $10.00 TryNow item. Using the below documentation a merchant can accurately represent totals and provide education to merchants.
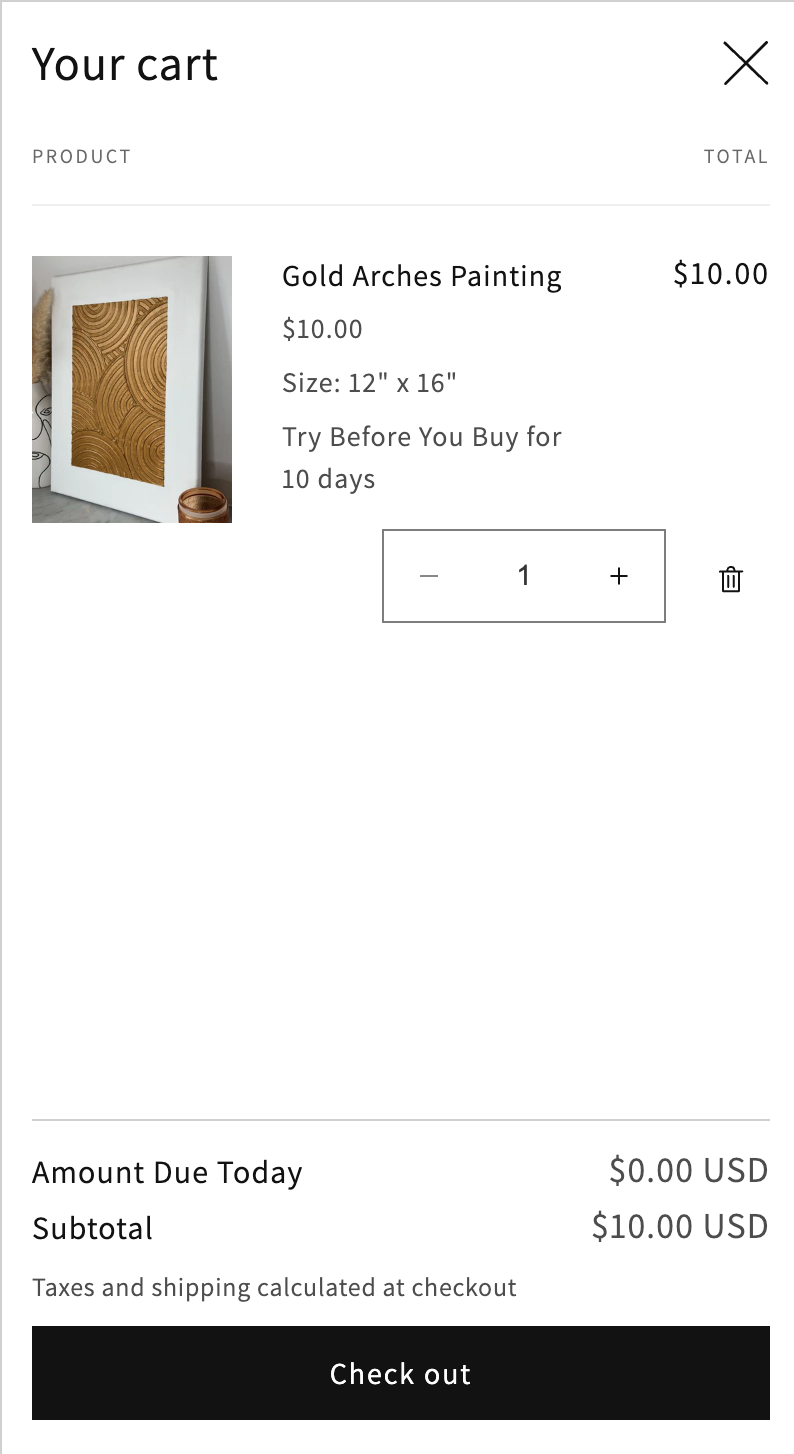
Prerequisites
Be able to add Try Before You Buy items to the cart. This will work with both mini carts and static cart pages.
Implementation - Amount Due
JavaScript
Some themes inject the mini-cart using Javascript. TryNow exposes a variety of methods to be able to handle cart features in these instances.
Implement in a few steps:
- Locate the file in which the change should be made. Likely a
cart-drawer.liquid
file or something similar. - Copy the following script, replacing
[[ELEMENT_TO_SWAP]]
with the selector of the element you'd like to render the TryNow Amount Due.<script type="text/javascript"> const cartObserver = new MutationObserver((mutationsList) => { mutationsList.forEach(async (record) => { if (!window.trynow) return; const tryNowItemCount = await window.trynow.cart.getTryNowItemCount() if (tryNowItemCount > 0) { await window.trynow.cart.replaceElementContentWithAmountDue(document.querySelector('[[ELEMENT_TO_SWAP]]')) } }) }) cartObserver.observe(document.body, { subtree: true, childList: true, }) </script>
- You may want to adjust the text of the
Subtotal
element to reflectAmount Due Today
if the cart is TryNow. To change that text, use the following snippet within the same<script>
tag as above. Replace[[SUBTOTAL_TEXT_ELEMENT
with the selector of the element you'd like to render the changed text.document.querySelector('[[SUBTOTAL_TEXT_ELEMENT]]').textContent = 'Amount Due Today'
- All together, you will have something like this:
<script type="text/javascript"> const cartObserver = new MutationObserver((mutationsList) => { mutationsList.forEach(async (record) => { if (!window.trynow) return; const tryNowItemCount = await window.trynow.cart.getTryNowItemCount() if (tryNowItemCount > 0) { await window.trynow.cart.replaceElementContentWithAmountDue(document.querySelector('[[ELEMENT_TO_SWAP]]')) document.querySelector('[[SUBTOTAL_TEXT_ELEMENT]]').textContent = 'Amount Due Today' } }) }) cartObserver.observe(document.body, { subtree: true, childList: true, }) </script>
replaceElementContentWithAmountDue()
defaults formatting toUSD
.
Rebuy Carts
TryNow Cart methods are compatible with Rebuy carts.
Update the element text and the subtotal text on cart change using the following snippet.
<script type="text/javascript">
async function useTryNowSubtotal() {
if (!window.trynow) return;
const tryNowItemCount = await window.trynow.cart.getTryNowItemCount()
if (tryNowItemCount > 0) {
await window.trynow.cart.replaceElementContentWithAmountDue(document.querySelector('[[ELEMENT_TO_SWAP]]'))
document.querySelector('[[SUBTOTAL_TEXT_ELEMENT]]').textContent = 'Amount Due Today'
}
}
// REBUY SPECIFIC LISTENERS
document.addEventListener('rebuy:cart.change', async (event) => {
await useTryNowSubtotal()
});
document.addEventListener('rebuy:cart.ready', async (event) => {
await useTryNowSubtotal()
});
document.addEventListener('rebuy:cart.add', async (event) => {
await useTryNowSubtotal()
});
document.addEventListener('rebuy:cart.enriched', async (event) => {
await useTryNowSubtotal()
});
</script>
Liquid
-
Locate where the subtotal is rendered to find placement of where the new TryNow subtotal should be added.
-
A variable should be assigned in Liquid to change this amount to reflect reductions based on TryNow items in the cart, i.e. the Amount Due Today. The calculations can be done based on the selling plan associated with the items in the cart.
<!-- TRYNOW --> <div class="totals" role="status"> <h2 class="totals__subtotal">{{ 'sections.cart.subtotal' | t }}</h2> {% assign amount_due_today = cart.total_price %} {% for item in cart.items %} {% if item.selling_plan_allocation and item.selling_plan_allocation.selling_plan.name contains 'Try Before You Buy' %} {% assign product_price = item.final_line_price %} {% assign amount_due_today = amount_due_today | minus: product_price %} {% endif %} {% endfor %} <p class="totals__subtotal-value">{{ amount_due_today | money_with_currency }}</p> </div> <!-- END TRYNOW -->
-
Test by adding products to the cart.
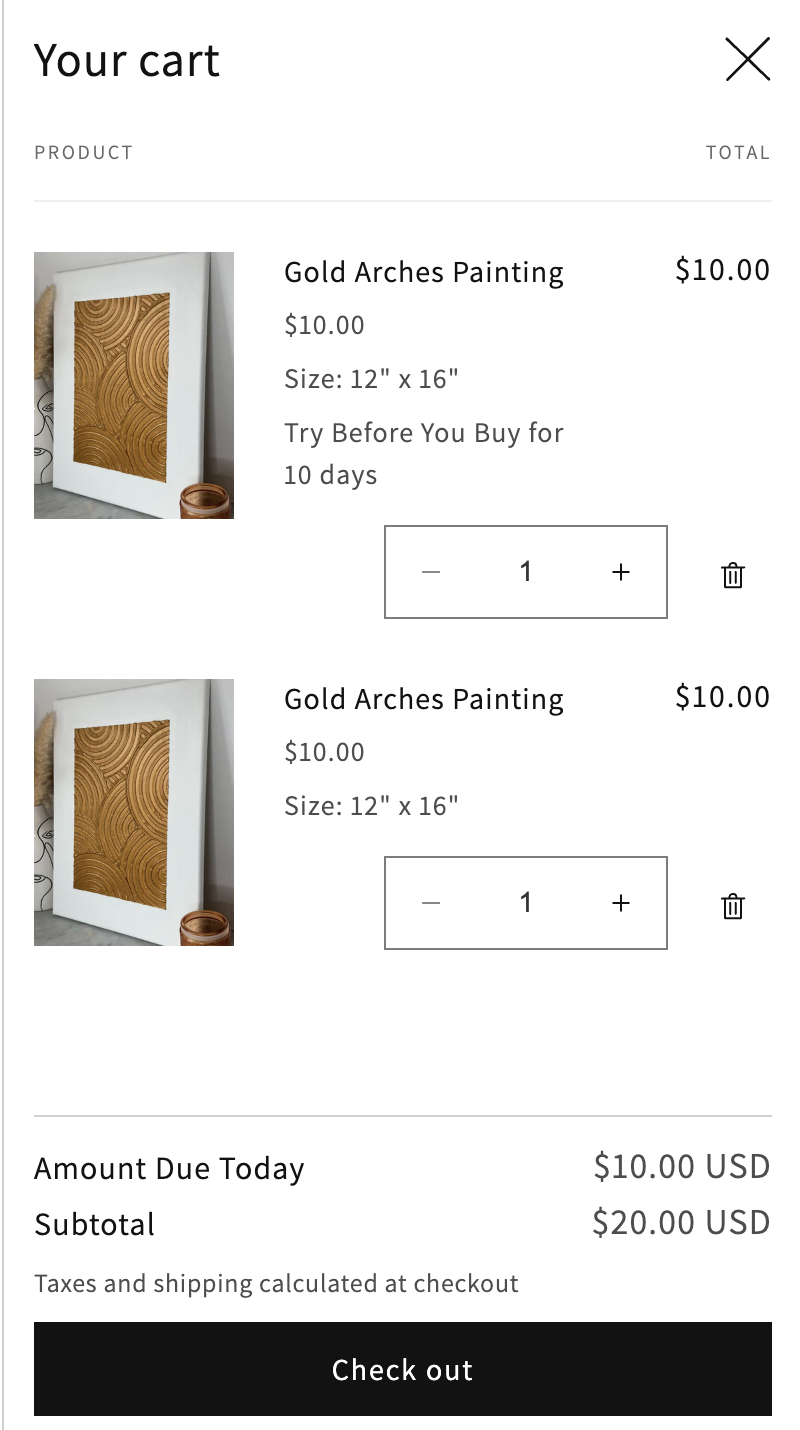
Implementation - Amount Charged if Kept
Amount charged if Kept
The Amount if Kept represents the value of the TryNow items all together.
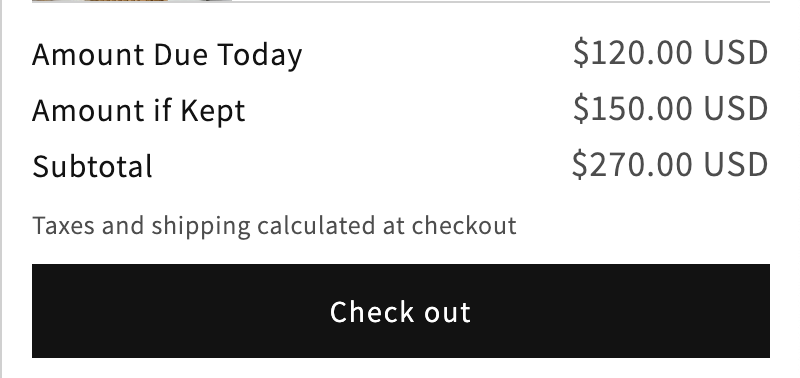
{% assign amount_if_kept = 0 %}
{% for item in cart.items %}
{% if item.selling_plan_allocation
and item.selling_plan_allocation.selling_plan.name contains 'Try Before You Buy'
%}
{% assign product_price = item.final_line_price %}
{% assign amount_if_kept = amount_if_kept | plus: product_price %}
{% endif %}
{% endfor %}
Additionally a second line of Liquid with the original total price can reflect how much the shopper will be charged if they keep everything in their cart.
{% if amount_due_today < cart.total_price %}
<p>Amount if kept — {{ cart.total_price }}</p>
{% endif %}
Hint
Match element types and classes to the styles you'd like to mirror.
Implementation - Authorization Warning
Authorization at Checkout
This will appear below the checkout button:
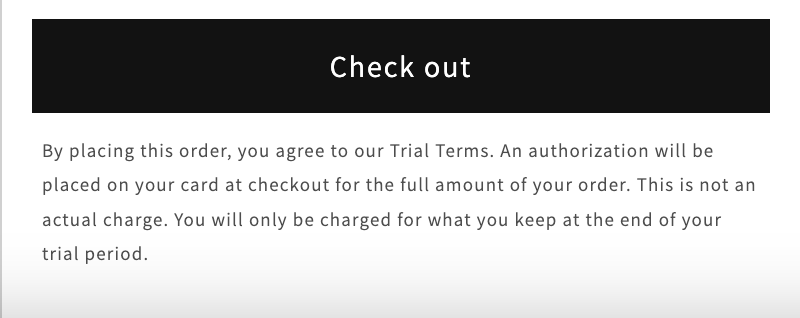
Adding some clarification text below the Checkout button will inform the shopper of what will happen to their credit card. Any styles and classes can be added to match the styles of your theme.
<p>
By placing this order, you agree to our Trial Terms. An authorization will be placed on your
card at checkout for the full amount of your order. This is not an actual charge. You will
only be charged for what you keep at the end of your trial period.
</p>
Troubleshooting
Editing Javascript cart
Depending on how your cart is built (e.g., minified JS), you might not be able to directly edit its markup. In that case you'll need to rely on DOM manipulation to append the element containing the 'Amount Due Today' value.
Updated 7 months ago