TryNow SDK Methods
Overview
The TryNow SDK provides integration with Shopify stores to enable try-before-you-buy functionality. It's accessible via the global window.trynow
object and offers methods for cart manipulation, product handling, and component management.
Using TryNow SDK Methods
In order to use methods from the TryNow SDK, you must have TryNow initialized in your theme. There are two ways to initialize TryNow.
Installation
The TryNow SDK is automatically initialized when the app embed is enabled in your Shopify theme. You can access it via the global window.trynow
object.
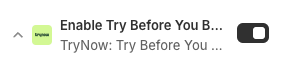
Initialization
You can also make the TryNow SDK available to you by using the initialization method.
// Basic initialization
window.trynow.initialize();
// Initialization with configuration
window.trynow.initialize({
myshopifyDomain: 'your-store.myshopify.com',
debug: true,
atcFailsafe: true
});
// Alternative static initialization
TryNow.initialize({
myshopifyDomain: 'your-store.myshopify.com'
});
Configuration Options
The SDK accepts the following configuration options:
Property | Type | Description | Default |
---|---|---|---|
myshopifyDomain | string | The Shopify domain of the store | window.Shopify?.shop |
storefrontAccessToken | string | The Storefront API access token (for headless mode) | undefined |
cartId | string | The cart ID if one exists already (for headless mode) | undefined |
debug | boolean | Enable debug mode for additional console logging | false |
waitForEvent | string | Custom event to wait for before initializing | undefined |
atcFailsafe | boolean | Enable Add to Cart Failsafe Monitor | false |
Core Properties
Property | Type | Description |
---|---|---|
cart | CartMethods | Methods for cart manipulation |
product | ProductMethods | Methods for product handling |
components | ComponentRegister | Access to component registration |
headless | boolean | Whether the SDK is running in headless mode |
initialized | boolean | Whether the SDK has been initialized |
config | TryNowInitConfig | Current configuration |
cartId | string | Current cart ID |
logger | Logger | Logger instance |
Methods
Configuration Methods
initialize(config?: TryNowInitConfig): TryNow
initialize(config?: TryNowInitConfig): TryNow
Initializes the TryNow SDK with the provided configuration.
window.trynow.initialize({
myshopifyDomain: 'your-store.myshopify.com',
debug: true
});
getConfig(): TryNowInitConfig
getConfig(): TryNowInitConfig
Gets the current configuration of the TryNow SDK.
const config = window.trynow.getConfig();
getMyshopifyDomain(): string
getMyshopifyDomain(): string
Gets the myshopify domain of the store.
const domain = window.trynow.getMyshopifyDomain();
getVersion(): string
getVersion(): string
Gets the SDK version.
const version = window.trynow.getVersion();
TryNow Functionality
getTrialPeriodDays(): Promise<number>
getTrialPeriodDays(): Promise<number>
Gets the number of trial period days.
window.trynow.getTrialPeriodDays().then(days => {
console.log(`Trial period: ${days} days`);
});
getSellingPlanId(): Promise<string>
getSellingPlanId(): Promise<string>
Gets the selling plan ID number as a string.
window.trynow.getSellingPlanId().then(id => {
console.log(`Selling plan ID: ${id}`);
});
hasPassedTryLink(): Promise<boolean>
hasPassedTryLink(): Promise<boolean>
Checks if the gated_link TryLink has been passed.
window.trynow.hasPassedTryLink().then(passed => {
if (passed) {
console.log('User has passed the TryLink gate');
}
});
addToCartClicked(): Promise<void>
addToCartClicked(): Promise<void>
Utility method to log TryNow CTA click analytics event. This is automatically called by TryNow CTA buttons.
// Only use this if manually creating a CTA button
customButton.addEventListener('click', () => {
window.trynow.addToCartClicked();
});
reset(): void
reset(): void
Clears and reloads product page data, refreshing all registered components.
window.trynow.reset();
Component Management
getComponent(id: string): HTMLElement | null
getComponent(id: string): HTMLElement | null
Gets a registered component by ID.
const component = window.trynow.getComponent('my-component-id');
defineComponents(): void
defineComponents(): void
Defines all TryNow custom web components.
window.trynow.defineComponents();
createVisibilityRuleset(rules: VisibilityRule[]): VisibilityRuleset
createVisibilityRuleset(rules: VisibilityRule[]): VisibilityRuleset
Creates a ruleset for controlling component visibility.
// Define the visibility rulesets
const ctaButtonRuleset = window.trynow.createVisibilityRuleset({
// example visibility rule:
rank: 10,
rules: [
{
rank: 0,
ruleTest: () => window.abTest === 'b',
ruleOutcome: () => {
const ctaButton = document.querySelector('#ctaButton');
if (ctaButton) {
ctaButton.style.display = 'block';
}
},
},
],
});
// Apply the rulesets to the components
window.trynow.getComponent('ctaButton', true).then(ctaButton => {
ctaButton.addRuleset(ctaButtonRuleset);
});
Utility Functions
waitForShopifyToLoad(): Promise<void>
waitForShopifyToLoad(): Promise<void>
Waits for Shopify to load before executing code.
window.waitForShopifyToLoad().then(() => {
// Code that depends on Shopify being loaded
});
Headless Mode
When initialized with a storefrontAccessToken
, the SDK operates in headless mode, which disables certain features that depend on the Shopify theme environment.
window.trynow.initialize({
myshopifyDomain: 'your-store.myshopify.com',
storefrontAccessToken: 'your-storefront-access-token',
cartId: 'existing-cart-id' // Optional
});
Events
The SDK dispatches a trynow:initialized
event when initialization is complete.
window.addEventListener('trynow:initialized', () => {
console.log('TryNow SDK has been initialized');
});
Error Handling
Most asynchronous methods return promises that should be handled with try/catch or .catch():
window.trynow.getTrialPeriodDays()
.then(days => {
console.log(`Trial period: ${days} days`);
})
.catch(error => {
console.error('Failed to get trial period days:', error);
});
Updated about 2 months ago